Table of Contents
How to test WCF service using svcutil?
First, compile your service into an executable file and include any dependent assemblies. Once you have these, head to the command prompt. You can then launch Svcutil.exe using the following format:
svcutil.exe /out:YourOutputFile.cs /config:YourConfigurationFile.config /service:YourServiceAddress
/out:YourOutputFile.cs: This parameter specifies the output file for the generated proxy class. This file will contain the client code that you’ll use to interact with your WCF service.
/config:YourConfigurationFile.config: This parameter tells Svcutil.exe where to find your service’s configuration file. The configuration file includes important details like endpoints, bindings, and behaviors.
/service:YourServiceAddress: This parameter indicates the address of your WCF service. This is where your client will connect to communicate with the service.
For more information about these parameters, check out the ServiceModel Metadata Utility Tool (Svcutil.exe) topic on the Microsoft website. It will help you explore the different options and give you a deeper understanding of how Svcutil.exe works.
Now, let me break down what happens when you run Svcutil.exe with these parameters:
1. Metadata Retrieval: Svcutil.exe contacts your WCF service at the specified address. It fetches the service’s metadata. Think of metadata as a blueprint of your service. It describes the service’s operations, data types, and other essential details.
2. Proxy Generation: Svcutil.exe uses the metadata it retrieved to generate a proxy class. This proxy acts as a middleman between your client application and the WCF service. It handles communication and simplifies interactions, letting you focus on your client logic instead of the low-level networking details.
3. Configuration File: Svcutil.exe can also create a configuration file based on the service’s metadata. This configuration file helps your client application connect to the WCF service correctly.
By running Svcutil.exe, you essentially generate the necessary code for your client to interact with your WCF service. This streamlined process makes it easy to test your service without needing to write all the communication code yourself.
What is SvcUtil.exe wsdl?
The dotnet-svcutil tool (often shortened to SvcUtil.exe) is a handy .NET tool that helps you easily interact with web services. Imagine it as a bridge between your application and a web service. It does this by retrieving metadata from a service, usually located on a network or stored in a WSDL file. This metadata tells your application everything it needs to know to talk to the web service.
Think of it this way: If the web service is a restaurant, SvcUtil.exe acts as a waiter, bringing you the menu (metadata). The menu lists all the dishes (web service operations) you can order (use). Using this menu, you can then order your food (call the web service operations) and get your meal delivered (receive the results).
SvcUtil.exe then uses this metadata to generate a WCF class, which is like a set of instructions that your application uses to communicate with the web service. This class contains client proxy methods that act as your application’s interface to the web service. With these methods, your application can easily access the web service operations, making it seamless to use the service’s functionality within your own code.
For example, if the web service provides a service for getting weather data, you might use SvcUtil.exe to generate a class containing methods like `GetWeatherForecast` and `GetCurrentTemperature`. Your application can then easily call these methods to get the weather data it needs.
How do you check WCF service is running or not?
To use the WCF Test Client, first select your service in Solution Explorer. You’ll typically find it with the extension .svc. Next, go to the Debug menu and choose Start Debugging (or simply press F5). Visual Studio will launch the WCF Test Client and run your code in debug mode. This gives you a great way to interact with your service directly and see if everything is working as expected.
Now, let’s dig a little deeper into what the WCF Test Client does. Think of it like a mini-application specifically designed for your WCF service. It lets you send requests to your service and see the responses it sends back. You can test different methods in your service, play around with different input parameters, and even inspect the data structures returned by the service.
There are a couple of things to remember about using the WCF Test Client for testing your WCF service:
It’s a debugging tool: While it’s great for verifying the service is up and running, it’s not a replacement for proper testing in a production environment. You’ll still want to use other tools and techniques to ensure your service is reliable and scales well.
Visual Studio is needed: This method is specifically for Visual Studio users. If you’re working with a different development environment, you might need to look at other tools to test your WCF service.
Remember, the WCF Test Client is a powerful tool in your WCF development toolbox. It makes it easy to check if your service is running correctly and to troubleshoot any issues you might encounter.
What is Svcutil exe to generate client code?
Svcutil.exe is a powerful tool that helps you generate client code for interacting with web services. Think of it as a translator that bridges the gap between your application and a web service. It takes a description of the service, called a WSDL (Web Services Description Language) or a policy file, and uses that information to create all the necessary code for your client application to communicate with the service.
Here’s how it works:
Receiving the Service Description: The first step involves providing Svcutil.exe with the WSDL or policy file. These files contain a detailed blueprint of the web service, outlining the operations it offers, the data it exchanges, and the protocols it uses.
Code Generation: Svcutil.exe then analyzes this service description and generates client-side code in your chosen programming language, typically C# or Visual Basic. This code includes classes, interfaces, and methods that mirror the functionality of the web service.
Client Application Interaction: Now, within your application, you can use the generated code to seamlessly interact with the web service. You can invoke its operations, send data, and receive responses, just like you would call methods within your own codebase.
In essence, Svcutil.exe acts as a bridge, allowing your application to “talk” to the web service without needing to understand the underlying technical details. It simplifies the process of consuming web services, enabling developers to focus on building their core application logic.
Let’s go back to the concept of UPNs (User Principal Names), which Svcutil.exe uses. An UPN is essentially a unique identifier for a user within a network or domain. The UPN combines the user’s account name with the domain name, separated by an “@” symbol. For instance, “[email protected]” represents the UPN for a user named “john.doe” in the domain “example.com.”
Svcutil.exe leverages UPNs in situations where authentication is required to access a web service. This allows for secure access to services, ensuring that only authorized users can interact with them.
What is the difference between Xsd exe and Svcutil exe?
Svcutil.exe is all about generating code for data contracts. Think of it as a translator between your XSD file and your code. If your XSD file supports data contracts, Svcutil.exe is your go-to tool. Data contracts make it easy for your code to work with XML data, and they’re perfect for web services.
But sometimes, an XSD file just doesn’t support data contracts. That’s where Xsd.exe steps in. It generates basic XML data types directly from your XSD file. This gives you a set of classes to work with the XML, but it’s not as “smart” as data contracts.
So, if you’re building a web service, try Svcutil.exe first. If you’re just working with XML data without needing data contracts, Xsd.exe is the tool for you.
Here’s a deeper dive into the difference between the two:
Svcutil.exe:
* Focuses on generating code for data contracts based on XSD files.
* Designed for building web services with XML data.
* Offers a more structured and streamlined way to interact with XML data.
Xsd.exe:
* Creates basic XML data types (classes) directly from XSD files.
* Works with any XSD file, even those that don’t support data contracts.
* Provides a simpler approach for handling XML data in general applications.
The choice between Svcutil.exe and Xsd.exe boils down to your specific needs. If you’re building a web service and your XSD file supports data contracts, Svcutil.exe is the clear winner. But if you just need to work with XML data in a more general context, Xsd.exe is a good alternative.
How to generate proxy for WCF service?
Manually, you can use the Service Model Metadata Utility Tool (SvcUtil.exe). This tool is a command-line utility that you can use to generate a client proxy from a WCF service’s metadata. To learn more about this tool, you can refer to the Microsoft documentation on the ServiceModel Metadata Utility Tool (SvcUtil.exe).
Within Visual Studio, you can use the “Add Service Reference” feature to generate a client proxy. This feature will automatically generate a proxy class that you can use to interact with the WCF service.
Let’s dive a bit deeper into how to generate a proxy using SvcUtil.exe. First, you need to obtain the metadata for the WCF service. This metadata can be in the form of a WSDL file or a MEX endpoint address. Once you have the metadata, you can use SvcUtil.exe to generate the proxy. The command will look something like this:
“`
svcutil.exe /out:MyProxy.cs /config:MyConfig.config /n:MyNamespace /t:code /reference:MyService.wsdl
“`
In this example:
`/out:MyProxy.cs`: Specifies the output file for the generated proxy class.
`/config:MyConfig.config`: Specifies the configuration file for the client proxy.
`/n:MyNamespace`: Specifies the namespace for the generated proxy class.
`/t:code`: Specifies that the output should be a code file (C# in this case).
`/reference:MyService.wsdl`: Specifies the WSDL file containing the service metadata.
After running the command, you’ll find a C# file containing the generated proxy class. You can then use this class to interact with the WCF service from your client application.
Using SvcUtil.exe gives you more control over the generated proxy, but it does require a bit of familiarity with command-line tools. If you prefer a more user-friendly approach, using the “Add Service Reference” feature in Visual Studio is a great option.
How to update connected services in Visual Studio?
First, expand the Connected Services node. Next, right-click the service reference you want to update. Then, select Update Service Reference.
But what exactly is happening when you update a service reference? Essentially, you’re telling Visual Studio to download the latest metadata from the service. This metadata contains information about the service, like the operations it offers, the data types it uses, and the way it communicates. When you update the service reference, Visual Studio takes this fresh metadata and uses it to update your project files. This ensures that your code is always in sync with the service and that you have access to all the latest features and functionalities. It’s kind of like updating your phone’s apps – it keeps everything running smoothly and gives you access to the latest features.
What is .net CLI?
Let’s break down what makes the .NET CLI so useful. Imagine it as your command center for everything .NET. It offers a suite of commands that let you manage your projects, build your applications, and deploy them to different environments. You can use it to create new projects, add packages, restore dependencies, build your code, run tests, and even publish your final application.
Think of it this way: the .NET CLI is like your trusty toolbox for building and managing .NET applications. It’s a consistent and reliable way to interact with your projects across different platforms, whether you’re working on Windows, macOS, or Linux. With the .NET CLI, you can streamline your development workflow and focus on what matters most – crafting innovative applications.
What is svcutil?
Think of it this way: You have a great new service you want to use in your project, but it’s written in a different language or uses a different format. svcutil steps in and helps you bridge that gap. It takes the information about the web service and generates a reference file that your project can understand. This reference file acts as a translator, letting your code communicate with the web service smoothly.
Here’s how it works: svcutil analyzes the web service’s metadata, which is like a description of its capabilities. This metadata could be in the form of a WSDL (Web Services Description Language) file or a similar format. Based on this metadata, svcutil creates a set of classes and interfaces. These classes and interfaces essentially represent the web service’s operations and data structures, making it easy for you to call the web service from your code.
Now, imagine trying to communicate with a foreign language speaker without a translator. You’d have a tough time! svcutil is like that translator, letting your code communicate fluently with web services written in different languages or using different protocols.
See more here: What Is Svcutil.Exe Wsdl? | The Dependent Tool Svcutil Exe Is Not Found
See more new information: musicbykatie.com
The Dependent Tool Svcutil.Exe Is Not Found: Troubleshooting Steps
So, what’s going on here? Well, svcutil.exe is a crucial tool. It’s the Windows Communication Foundation (WCF) command-line utility. Think of it like a Swiss Army knife for working with web services. It helps you generate proxy classes, which are basically like translators that let your application talk to web services.
The error message “the dependent tool svcutil.exe is not found” means that your project can’t find svcutil.exe. There are a few likely culprits:
1. You’re Missing the .NET Framework SDK: This is the most common culprit. The svcutil.exe tool is part of the .NET Framework SDK. So, if you haven’t got it installed, you’ll get this error.
2. Incorrect Path: Even if you’ve got the SDK installed, the path to svcutil.exe might not be set up correctly in your system’s environment variables. Think of environment variables like a directory map for your computer. If the path is wrong, your system can’t find svcutil.exe.
3. Conflicting Versions: If you have multiple versions of the .NET Framework installed, you might have a version conflict. You could be using an older version of svcutil.exe that’s not compatible with your project.
Here’s a step-by-step guide to fix that annoying error:
Step 1: Check Your .NET Framework SDK:
* Go to the Control Panel.
* Click on Programs and Features.
* Look for .NET Framework SDK. If you see it, you’re good. If not, you need to install it.
Step 2: Download and Install the .NET Framework SDK:
* Head to the [Microsoft website](https://dotnet.microsoft.com/download/dotnet-framework).
* Grab the latest version of the .NET Framework SDK.
* Follow the on-screen instructions to install it.
Step 3: Set Up Environment Variables (If Needed):
* Windows: Press Windows Key + R, type sysdm.cpl and hit Enter.
* Click on the Advanced tab, then click Environment Variables.
* Under System variables, find the Path variable.
* Click Edit, then click New.
* In the new entry, paste the path to your svcutil.exe directory. (Usually, it’s something like C:\Program Files (x86)\Microsoft SDKs\Windows\v10.0A\bin\NETFX 4.8 Tools ).
* Click OK to save the changes.
Step 4: Restart Your Computer:
* This might seem obvious, but it’s important to restart your computer after making environment variable changes. This makes sure the changes take effect.
Step 5: Try to Generate Your Proxy Classes Again:
* Open up your Visual Studio project and try generating your proxy classes again. If everything’s set up correctly, the error should be gone.
What If You’re Still Stuck?
* Check Your Project’s Settings: Make sure your project is set up to use the correct version of the .NET Framework. If you’re using a project that was built with an older version, you might need to update it.
* Look for Conflicts: If you have multiple versions of the .NET Framework installed, try uninstalling the older ones to see if that resolves the issue.
* Do a Clean and Rebuild: Try cleaning and rebuilding your solution. This can sometimes help with unexpected issues.
* Search Online: If you’re still stuck, search online for more specific solutions related to your project or the type of web service you’re working with.
FAQs:
* What if svcutil.exe is still not found after following these steps?
* Double-check that you entered the correct path in your environment variables. Make sure there are no typos.
* Run the Visual Studio Command Prompt (VS2022 Developer Command Prompt) as administrator.
* Is svcutil.exe the only way to generate proxy classes?
* No, there are other ways to generate proxy classes, like using the Add Service Reference option in Visual Studio.
* Why do I need to generate proxy classes?
* Proxy classes act as a bridge between your application and the web service. They handle the communication details, making it easier for your application to interact with the service.
* What are some common causes of this error?
* Missing or outdated .NET Framework SDK
* Incorrect environment variable settings
* Conflicting versions of the .NET Framework
This whole process might sound daunting, but don’t worry. Most of the time, it’s a simple fix. Just follow these steps, and you’ll be back up and running with your web services in no time!
windows – svcutil.exe is not recognized as an internal or external …
The ServiceModel Metadata Utility Tool can be found at the Windows SDK installation location, specifically, C:\Program Files\Microsoft SDKs\Windows\v6.0\Bin. Super User
Troubleshoot the Get started with Windows Communication
Use the Svcutil.exe tool ‘Svcutil’ is not recognized as an internal or external command, operable program, or batch file. Svcutil.exe must be in the system path. The Microsoft Learn
How to: Use Svcutil.exe to Validate Compiled Service Code
You can use the ServiceModel Metadata Utility Tool (Svcutil.exe) to detect errors in service implementations and configurations without hosting the service. To Microsoft Learn
cannot install dotnet-svcutil · Issue #3816 · dotnet/wcf ·
When I attempt to install dotnet-svcutil using “Extensions and Updates” in Visual Studio, I get these errors: Severity Code Description Project File Line Github
how-to-use-svcutil-exe-to-download-metadata-documents.md
You can use Svcutil.exe to download metadata from running services and to save the metadata to local files. For HTTP and HTTPS URL schemes, Svcutil.exe attempts to Github
svcutil dosen’t work with .NET6 · Issue #4766 · dotnet/wcf
The version with .NET6 support is finally released, you can try out by reinstalling the tool with commandlines below. Uninstall existing version: dotnet tool github.com
WCF svcutil tool overview – .NET | Microsoft Learn
The Windows Communication Foundation (WCF) dotnet-svcutil tool is a .NET tool that retrieves metadata from a web service on a network location or from a microsoft.com
Error : The dependent tool ‘svcutil.exe’ is not found.
I know this is very old, but I just found it and think there’s no need to change the current version of your SDK, the only thing you need is copy the svcutil.exe from the microsoft.com
How To Solve Signtool.Exe Not Found In Visual Studio 2015
How To Solve Signtool.Exe Not Found In Visual Studio 2015.
How To Fix Windows Cannot Find Appdata Local Temp Subfolder Filename.Exe On Startup Error
\”The System Can Not Find The File Specified\” – Visual Studio Error Fixed.
How To Test Wcf Service Using Wcftestclient
Fix Error Windows Cannot Find C:\\Program Files\\Microsoft Office\\Root\\Office16\\Winword.Exe
Proxy Class Or Dll File From Wsdl File Using Wsdl Exe Using Command Prompt
Not Showing Solution Explorer Visual Studio 2022
Link to this article: the dependent tool svcutil exe is not found.
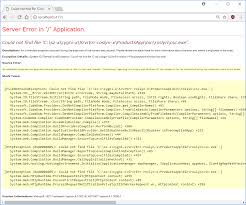
See more articles in the same category here: https://musicbykatie.com/wiki-how/