Table of Contents
What is the queueable class in Apex Salesforce?
Queueable allows you to put these lengthy processes in a queue, and Salesforce will then execute them asynchronously in the background. This means your users don’t have to wait, and the process can run without impacting the performance of your application.
Think of it like placing an order at a restaurant. You don’t have to wait in the kitchen while your food is being prepared; you get a number and wait comfortably. Similarly, Queueable lets you schedule your complex processes and get on with other tasks while Salesforce handles the execution in the background.
Here are some key benefits of using the Queueable interface:
Improved performance: By offloading long-running tasks to the background, you can ensure that your application remains responsive and doesn’t experience performance issues.
Enhanced scalability:Queueable allows you to process large volumes of data without impacting the performance of your application.
Increased reliability:Queueable allows you to run processes that might require multiple steps or interact with external systems, ensuring that the entire process is completed successfully even if there are temporary errors or delays.
Simplified development:Queueable provides a simple and straightforward way to implement asynchronous operations in your Apex code. You can define your process logic in a separate class and then simply queue it for execution.
Queueable is a valuable tool for building robust and scalable Salesforce applications. It allows you to perform complex operations efficiently, without impacting user experience, and ensures the reliability and stability of your code.
What is the difference between batch apex and Queueable apex in Salesforce?
Batch Apex shines when you need to process large volumes of data. Imagine you’re updating thousands of records—Batch Apex steps in to handle this efficiently. It divides your data into manageable chunks called “batches,” allowing you to process them in parallel. This parallel processing significantly speeds up your operations. However, Batch Apex limits you to a maximum of five simultaneous tasks.
Queueable Apex is perfect for smaller, more focused jobs. Think of it as a more flexible, on-demand worker. While Batch Apex requires you to define specific logic for batch processing, Queueable Apex offers more freedom to handle various tasks. It doesn’t have the batching limitations of Batch Apex, allowing you to run up to 100 simultaneous tasks.
So, how do you choose between the two? If you’re dealing with massive amounts of data, Batch Apex is your go-to. Its parallel processing capabilities make it ideal for bulk updates, deletions, or complex calculations. But if you need to handle smaller, more independent tasks, Queueable Apex gives you greater flexibility and control.
Here’s a simple analogy: Imagine you need to clean your house. If you have a massive cleaning job, you might break it down into smaller tasks like cleaning each room. That’s like Batch Apex—you’re dividing your work into manageable chunks. But if you just need to do a quick tidy-up of a small area, you’d tackle it directly. That’s like Queueable Apex—it’s for quick, focused tasks.
How many numbers of jobs can I queue using system enqueueJob() at a time?
It’s important to understand the limitations of System.enqueueJob. While it allows you to queue jobs efficiently, there’s a limit on how many jobs you can process at once. This limit ensures that your Salesforce org remains stable and performs well. It’s important to consider your use case and make sure you’re not exceeding the limit.
Here’s how to think about it:
Think of each job as a task: Imagine you’re organizing your day and need to complete several tasks. You can only handle a certain number of tasks at a time without getting overwhelmed.
System.enqueueJob as a task manager: System.enqueueJob acts like a task manager for your Salesforce org. It helps you queue up jobs for processing later, but it has a limit to ensure your org doesn’t get overloaded.
How can you use System.enqueueJob effectively?
Break down large tasks: If you have a large process to complete, break it down into smaller, manageable tasks. Then, you can use System.enqueueJob to queue up these smaller tasks.
Prioritize tasks: If you have multiple tasks, prioritize them. Use System.enqueueJob to queue up the most important tasks first.
Monitor your job queue: Keep an eye on your job queue to ensure that jobs are processing efficiently. If you notice a buildup of jobs, you may need to adjust your code or processes.
By following these tips, you can effectively use System.enqueueJob to improve your application’s efficiency and scalability.
How to call queueable?
To execute a queueable apex, you first need to implement the Queueable interface. This interface makes your class ready to be queued for execution later. Think of it like putting a task in a queue, ready to be processed when the system has time.
Secondly, ensure your method that implements the Queueable interface is declared as either public or global. This lets you access and call the method from different parts of your Salesforce org.
Finally, if your queueable apex needs to make external calls (like to a web service), you’ll need to implement Database.AllowsCallouts. This is crucial to allow your queueable apex to communicate with other systems.
Let’s break down a simple example to illustrate how all this works:
“`java
public class MyQueueable implements Queueable {
public void execute(QueueableContext context) {
// Your code to be executed later goes here
System.debug(‘Queueable is running!’);
}
}
“`
In this example, we have a class called `MyQueueable` that implements the `Queueable` interface. The `execute` method is where you put your code that will run later. The `QueueableContext` object provides information about the current context of execution.
Now, to actually call this queueable, you can use the `System.enqueueJob` method:
“`java
MyQueueable myQueueable = new MyQueueable();
Id jobId = System.enqueueJob(myQueueable);
“`
This code creates a new instance of `MyQueueable` and enqueues it. `jobId` will hold the ID of the job that’s been queued for execution.
Remember: Queueable jobs are executed asynchronously, which means they will run in the background. This is ideal for tasks that are time-consuming or don’t require immediate results.
I hope this gives you a better understanding of how to call queueable apex. Let me know if you have any more questions!
Can we call Queueable apex from batch?
Queueable Apex lets us split a task into smaller chunks, then send those chunks off to run in the background. It’s a great option when you need to do a lot of work without holding up your users. You can use Queueable Apex from a Future method too, giving you even more flexibility.
Batch Apex, on the other hand, is for working with large datasets. It lets us process data in batches, and that’s often a much more efficient way to handle those big jobs.
So, can you call Queueable Apex from Batch? The answer is yes, but there’s a little more to it. Let’s dive into the details:
Why you might want to use Queueable Apex from a Batch Apex job:
Breaking down a complex process: Imagine you’re processing a large amount of data. You could use Batch Apex to break it into smaller chunks, and then within each batch, use Queueable Apex to perform even finer-grained operations. This allows you to distribute the work across multiple transactions, making it more manageable and improving performance.
Handling asynchronous dependencies: Sometimes a Batch Apex job needs to trigger some asynchronous tasks. Queueable Apex is a perfect way to handle this, as it allows you to queue these tasks for later execution without blocking the main Batch Apex process.
Let’s look at a common use case:
1. You have a large list of leads to process.
2. You use Batch Apex to split this list into smaller batches.
3. For each batch, you use a Queueable Apex class to perform more complex actions like sending emails, updating records, or integrating with external systems.
This approach keeps the overall processing time down, reduces the risk of hitting governor limits, and ensures a smoother user experience.
Important Considerations:
Governor Limits: Queueable Apex also has its own governor limits, so be mindful of how many jobs you’re queuing within a single transaction.
Error Handling: You’ll want to implement robust error handling for both your Batch Apex and Queueable Apex classes to ensure that jobs complete successfully, even if an error occurs.
Transaction Control: Remember that each Queueable Apex job runs in its own transaction, so be aware of the implications for data consistency.
Queueable Apex in Developer Edition: The Developer Edition of Salesforce has limits on the number of Queueable Apex jobs you can execute.
By combining the power of Batch Apex with the flexibility of Queueable Apex, you can tackle complex tasks in Salesforce efficiently and effectively.
What is the difference between future method and Queueable apex?
Future methods allow you to execute code asynchronously, meaning it runs in the background and doesn’t block the user interface. However, a Future method only handles one asynchronous transaction at a time.
Queueable Apex takes things a step further by enabling you to process data in chunks, making it ideal for large datasets. Think of it as a conveyor belt for your code. You can divide your data into smaller batches, process each batch with a Queueable Apex job, and chain these jobs together to complete the overall task. This allows you to spread out the processing workload and prevent hitting Apex limits.
A key advantage of Queueable Apex is its Finalizer feature. Finalizers give you the power to reschedule a job if it encounters a limit exception. This ensures your job gets completed, even if it faces temporary roadblocks.
Here’s a more detailed explanation:
Imagine you have a large list of customers that you need to update in Salesforce. With a Future method, you could process one customer at a time. But, if you have thousands of customers, this could take a long time and potentially cause your Apex code to timeout.
Queueable Apex allows you to split the customer list into smaller groups and process each group in a separate job. You can then chain these jobs together, ensuring that all customers are eventually updated. If one job hits a limit exception, the Finalizer allows you to reschedule that job, ensuring that the processing continues until all customers are updated.
In short, Queueable Apex provides you with more flexibility and control over your asynchronous processes, making it a powerful tool for handling large datasets and complex tasks.
See more here: What Is The Difference Between Batch Apex And Queueable Apex In Salesforce? | Queueable Apex Class In Salesforce
What is the difference between future and queueable apex in Salesforce?
Let’s dive into the key differences between Future and Queueable Apex in Salesforce. These two powerful tools let you run long-running processes asynchronously, preventing your user interface from getting bogged down.
Future Apex is a simple and efficient way to execute a method in the background. It’s perfect for quick tasks that don’t require much processing time. Queueable Apex, on the other hand, is a more robust solution for complex jobs that might involve multiple steps or interactions with external systems.
Here’s a breakdown of their core differences:
Future Apex:
* Uses the `@future` annotation, directly applied to the method you want to execute asynchronously.
* This makes it easy to implement, especially for quick tasks.
* The limitations lie in its ability to only execute one method.
* It is also limited in terms of error handling, as it doesn’t allow for retries or custom error management.
Queueable Apex:
* Requires implementing the `Queueable` interface in your Apex class.
* This approach allows you to manage complex tasks with multiple steps or interactions.
* It offers more control and flexibility with error handling, providing options for retries and custom error management.
Let’s illustrate this with an example:
Imagine you need to update thousands of records in Salesforce. With Future Apex, you might split the process into smaller batches and use a `@future` method to update each batch. However, you won’t have much control over how the process handles errors or if a batch fails to update.
Queueable Apex provides a much more powerful solution in this scenario. You could create a `Queueable` implementation that:
1. Reads the records to be updated in batches.
2. Processes each batch asynchronously using a `@future` method.
3. Handles any errors that occur during processing, potentially retrying failed updates or logging detailed error information.
This example highlights the flexibility and robustness of Queueable Apex, making it the preferred choice for complex tasks.
How to implement queueable apex?
To get started with Queueable Apex, you need to create a few key components. These are:
A generic job object (AsyncJob.cls) This is the heart of your Queueable Apex implementation. It’s where you define the actual job that needs to be executed asynchronously. Think of it as the blueprint for your asynchronous task.
A callable class for method configuration (GenericCallable.cls) This class helps you configure the methods that will be executed as part of your asynchronous job. It’s like the instruction manual for your job.
A service class for the orchestration of these elements (AsyncJobService.cls) This is the conductor of your Queueable Apex symphony. It brings together the job object and the callable class to execute your asynchronous task in an organized manner.
Now, let’s go through a step-by-step guide to help you understand how to put all these pieces together:
1. Creating the AsyncJob.cls (The Job Blueprint)
Start by creating a class called AsyncJob that implements the Queueable interface. This interface ensures that your class can be queued and processed asynchronously. Inside this class, you’ll define a method called execute, which is the method that will be executed when the job is dequeued. This method is the core of your asynchronous logic.
“`java
public class AsyncJob implements Queueable {
// … Your job specific data …
public void execute(QueueableContext context) {
// Implement your asynchronous logic here.
// For example, you might process a large data set,
// send an email, or update records.
}
}
“`
2. Creating the GenericCallable.cls (The Method Configuration)
Next, create a class called GenericCallable to configure and manage your callable methods. This class should implement the Callable interface. The purpose of this class is to provide a flexible way to call various methods based on your specific needs.
“`java
public class GenericCallable implements Callable {
// … Your job specific data …
public Object call() {
// … Your job specific logic to call methods …
}
}
“`
3. Creating the AsyncJobService.cls (The Orchestrator)
The final piece of the puzzle is the AsyncJobService class. This class acts as the central control point for your asynchronous jobs. It will handle tasks like creating and enqueuing the AsyncJob instances, managing the execution of these jobs, and handling any errors that might occur.
“`java
public class AsyncJobService {
public static void enqueueJob(AsyncJob job) {
// Code to enqueue the AsyncJob
Queue.enqueue(job);
}
// … Other methods for managing and orchestrating your jobs …
}
“`
By using this service class, you can efficiently manage and execute your asynchronous tasks. It provides a structured and reusable approach to handling Queueable Apex jobs.
Understanding the Process
Now that we’ve created the key elements, let’s see how this all works together.
1. Job Creation: First, you’ll create an instance of your AsyncJob class. This instance will contain the specific data and logic needed for your asynchronous task.
2. Job Enqueuing: You’ll then use the AsyncJobService class to enqueue your job. This involves adding the job to the Salesforce asynchronous job queue.
3. Job Dequeuing and Execution: When a worker thread becomes available in the Salesforce platform, it will dequeue your job from the queue and execute the execute method of your AsyncJob class.
4. Completion: Once the execute method completes its execution, the job is considered finished.
Benefits of Queueable Apex
By implementing Queueable Apex, you can take advantage of several benefits:
Improved Performance: Long-running tasks don’t block your user interface, ensuring smooth operation for users.
Scalability: You can process large amounts of data or perform complex tasks without impacting system performance.
Flexibility: Queueable Apex allows you to easily schedule and manage asynchronous operations.
Keep in mind that this is a basic framework for Queueable Apex implementation. There are many ways to extend and customize this approach to fit your specific needs. For instance, you could add more advanced error handling, logging, or data management capabilities.
How to enqueue apex job in Salesforce?
But what happens next? Here’s the breakdown:
1. Scheduling: Salesforce puts your Queueable Apex job on a queue. This queue is like a waiting list for jobs to be processed.
2. Execution: When Salesforce has some free resources, it takes your job from the queue and runs it.
3. Progress Update: As your job runs, it might update records, send emails, or perform other tasks.
4. Completion: Once your job finishes its work, it’s removed from the queue.
This process ensures your job runs smoothly without impacting the performance of your users’ interactions with Salesforce.
Here’s why using Queueable Apex is beneficial:
Scalability: Your job can be processed efficiently even when many users are working in Salesforce, as it doesn’t block other requests.
Performance: You’re not limited by the time allowed for a single transaction, so you can perform complex tasks without exceeding the standard transaction limits.
Flexibility: You can schedule jobs to run at specific times or based on certain events.
Reliability: The Queueable Apex mechanism ensures your job runs to completion, even if the user who initiated it leaves Salesforce.
With Queueable Apex, you can build sophisticated and efficient automation workflows in Salesforce. Let me know if you’d like to explore specific examples or dive deeper into any of these aspects!
What is a queueable apex class?
The heart of a Queueable Apex class is the `execute` method. This method is the entry point for your asynchronous job and contains the code that you want to execute in the background. You can think of the `execute` method as the main function of your asynchronous task.
To create a Queueable Apex class, you need to implement the `Queueable` interface. This interface defines the `execute` method, which is required for all Queueable Apex classes.
Here’s how to implement a basic Queueable Apex class:
“`java
public class AsyncJob implements Queueable {
private GenericCallable toExecute;
public AsyncJob(GenericCallable toExecute) {
this.toExecute = toExecute;
}
public void execute(QueueableContext context) {
// Execute the logic of your asynchronous job here
toExecute.execute();
}
}
“`
In this example, we have a class called `AsyncJob` that implements the `Queueable` interface. It takes a `GenericCallable` object in its constructor, which represents the actual task you want to execute. The `execute` method then calls the `execute` method of the `GenericCallable` object to perform the asynchronous task.
`Queueable Apex` classes are great for tasks that involve:
* Sending emails to a large number of contacts
* Importing data from external sources
* Processing large amounts of data
* Performing calculations that take a long time to complete
Queueable Apex classes allow you to build robust and scalable applications that can handle complex asynchronous tasks with ease. This can significantly improve the performance and responsiveness of your Salesforce applications.
Here’s a deeper look at how Queueable Apex classes work under the hood:
1. Submitting the Job: When you submit a Queueable Apex job, the platform creates a new job record and adds it to a queue. This queue is managed by Salesforce and ensures that your jobs are processed in an efficient and reliable manner.
2. Processing the Job: The platform’s job scheduler periodically checks the queue for jobs that are ready to be processed. When your job is selected, it is executed in a separate thread. This means that your job runs in the background, without blocking the user’s interaction with the platform.
3. Handling Errors: If your job encounters an error, the platform will log the error and attempt to retry the job. This ensures that your jobs are completed successfully, even if they encounter unexpected issues.
By using Queueable Apex, you can design and develop Salesforce applications that are more efficient, reliable, and scalable.
See more new information: musicbykatie.com
Queueable Apex Class In Salesforce: A Comprehensive Guide
Alright, so you’re working with Salesforce and you need to process a big chunk of data, maybe update a bunch of records or send out a ton of emails. You could do it all at once, but that might slow your Salesforce org down. What’s a developer to do? Queueable Apex, my friend! It’s your secret weapon for smooth, efficient batch processing.
Think of it like this: Imagine you’re at the grocery store and have a giant cart overflowing with items. Would you try to check out all at once? Probably not. You’d likely split your cart into smaller, more manageable batches, right? Queueable Apex works the same way, dividing your massive tasks into smaller chunks that your Salesforce org can handle without breaking a sweat.
Let’s Get Specific:
Queueable Apex is a powerful Salesforce feature that lets you execute long-running processes in the background, without blocking the user interface. Think of it as a way to handle your data like a pro, without interrupting your users.
Here’s how it works:
1. You create a class that implements the `Queueable` interface. This class defines the logic for the tasks you want to process.
2. You create a `QueueableJob` object in Salesforce, which stores information about your queued process. This includes things like the class you want to execute and any data you need to pass to it.
3. The `System.enqueueJob` method kicks off the process, placing it in the queue. Salesforce then handles executing the queued job asynchronously, meaning it runs in the background without blocking user interactions.
4. Your queued job is processed in batches. This helps prevent performance issues and ensures that your long-running tasks are handled efficiently.
Why is this a big deal?
Improved Performance: Queueable Apex helps prevent your Salesforce org from getting bogged down with long-running tasks. By processing data in batches, it ensures that your users have a smooth and responsive experience.
Asynchronous Execution: This lets you execute your tasks in the background without impacting user interactions. So, you can continue working on other tasks while your queued job runs its course.
Error Handling: Queueable Apex provides a way to handle errors gracefully. If your job encounters an issue, you can capture it, log it, and potentially retry the failed batch.
Scalability: This is perfect for handling large volumes of data, making it a great choice for batch updates, mass email campaigns, and other data-intensive tasks.
Ready to Dive In?
Here’s a simple example of a Queueable Apex class:
“`java
public class AccountUpdateJob implements Queueable {
public void execute(QueueableContext context) {
List
// Add Accounts to the list
// Update the Accounts
// Save the updated Accounts
}
}
“`
Let’s break this down:
1. The `AccountUpdateJob` class implements the `Queueable` interface. This tells Salesforce that this class is designed to be run in a queued fashion.
2. The `execute` method is the heart of the job. It’s where you write the logic for updating your accounts.
3. The `QueueableContext` object provides information about the current job execution. This can be useful for logging or error handling.
To execute this job, you would use the following code:
“`java
AccountUpdateJob job = new AccountUpdateJob();
Id jobId = System.enqueueJob(job);
“`
This code does the following:
1. Creates a new `AccountUpdateJob` instance.
2. Uses `System.enqueueJob` to queue the job.
3. Stores the job ID in the `jobId` variable. You can use this ID to check on the status of the job or to access its execution details.
Pro Tips:
Use the `QueueableContext` object to access information about the job execution. This can be helpful for logging, error handling, and for identifying the specific batch being processed.
Consider using the `@InvocableMethod` annotation to create Queueable methods from your Apex controller classes. This can make it easier to create and manage Queueable jobs.
Implement error handling in your `execute` method. This will help you identify and resolve issues that may occur during job execution.
Use the `System.schedule` method to schedule your Queueable jobs to run at specific times or intervals. This can be useful for automating tasks or for running jobs at specific points in time.
That’s the Basics!
Queueable Apex is an invaluable tool for any Salesforce developer who needs to handle large-scale processes efficiently. With this knowledge, you can unlock its power and streamline your data operations with ease.
FAQs:
Q: What are some real-world examples of how Queueable Apex can be used?
A: Here are a few examples:
Batch Updating Records: Use Queueable Apex to update large numbers of records, such as changing the status of a set of opportunities or updating customer contact information.
Mass Email Campaigns: Send out emails to a large number of contacts or leads without overloading your Salesforce org.
Data Migrations: Move data between different objects or systems, ensuring a smooth and efficient transfer.
Data Cleansing: Identify and correct errors in your data, improving the accuracy and integrity of your information.
Q: Is there a limit to the number of Queueable jobs I can execute at once?
A: Yes, there are limits. The specific limits depend on your Salesforce edition and organization size. You can check the Salesforce documentation for the exact limits applicable to your org.
Q: What happens if a Queueable job fails?
A: Salesforce provides error handling mechanisms for Queueable Apex jobs. If a job fails, you can capture the error, log it, and potentially retry the failed batch. This helps ensure that your data is processed accurately and that any errors are handled appropriately.
Q: Can I use Queueable Apex to run scheduled jobs?
A: Yes! You can use the `System.schedule` method to schedule your Queueable jobs to run at specific times or intervals. This allows you to automate tasks, such as running daily reports or updating data regularly.
Queueable Apex | Apex Developer Guide | Salesforce
Take control of your asynchronous Apex processes by using the Queueable interface. This interface enables you to add jobs to the queue and monitor them. Using the interface is an enhanced way of running your asynchronous Apex code compared to using future Salesforce Developers
Queueable Interface | Apex Reference Guide
To execute Apex as an asynchronous job, implement the Queueable interface and add the processing logic in your implementation of the execute method. To implement the Salesforce Developers
Understand Queueable Apex with Example and Test
Create a Queueable Apex class that inserts the same Contact for each Account for a specific state. Write unit tests that Salesforce Bolt
Control Processes with Queueable Apex | Salesforce Trailhead
Queueable Apex allows you to submit jobs for asynchronous processing similar to future methods with the following additional benefits: Non-primitive types: Your Queueable Trailhead
Queueable Apex in Salesforce – The Ultimate Guide
Queueable Apex, as a feature in Salesforce, introduces a more robust and flexible way to run asynchronous Apex code. It complements the existing functionality of saasguru
Generic Approach to Salesforce Queueable – Apex Hours
Summary. Technical Overview. This implementation simply consists of three parts. A generic job object, AsyncJob.cls. A callable class for class/method configuration, GenericCallable.cls. A service class for Apex Hours
Queueable Apex in Salesforce with example – Salesforce Geek
In this blog, we are going to discuss Queueable Apex in Salesforce with example. Queueable Apex is another asynchronous type of apex. It runs in a separate Salesforce Geek
Queueable Apex | Apex Developer Guide | Salesforce
Apr 8, 2020. Interviewer: What is Queueable Apex? Interviewee: It’s an extension of the Future Methods. It uses the Queueable interface which is an enhanced way of running your asynchronous… Medium
Future Vs Queueable Apex – Apex Hours
Salesforce Developer. Future Vs Queueable Apex. Prakash Jada. Mar 17, 2023. 15 Comments. What is the difference between future method and Queueable Job in Apex? Join us to learn Apex Hours
Queueable Vs Batch Apex In Salesforce – SalesforceCodex
Queueable apex can be called from the Future and Batch class. In Queueable Apex, we can chain up to 50 jobs and in the developer edition, we can chain salesforcecodex.com
Queueable Apex In Salesforce
Salesforce – Queueable Apex
Control Processes With Queueable Apex || Asynchronous Apex || Salesforce
06 Example – Queueable Apex Implementation With Test Class | Asynchronous Apex In Salesforce
Day 3 : Queueable Apex | Asynchronous Apex | Salesforce #Salesforce #Apex #Apexlegends#Asynchronous
Async Apex In Salesforce | Queueable Apex With Example | Asynchronous Apex
Queueable In Salesforce – Asynchronous Apex Part – 2
Asynchronous Apex Using Future Method And Queueable Apex
Link to this article: queueable apex class in salesforce.
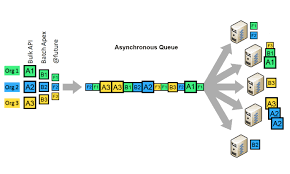
See more articles in the same category here: https://musicbykatie.com/wiki-how/