Table of Contents
How do you define comp-3 in COBOL?
COMP-3 represents a packed decimal field, which means each byte holds two decimal digits, making the most of available storage space. The last byte, however, has a special role – it acts as the sign field.
Here’s how the sign field works:
x’C’: This value represents a positive number.
x’D’: This value indicates a negative number.
x’F’: This value signifies an unsigned number.
In addition to the sign, COMP-3 fields are defined with a specific number of digits and an implied decimal point. For example, a COMP-3 field defined as PIC S9(5)V99 would have five digits to the left of the decimal point and two to the right.
Why is COMP-3 so special?
COMP-3 offers a unique blend of benefits:
Efficiency:COMP-3 fields are compact, storing two digits per byte, which helps conserve memory, especially for large datasets.
Precision: COMP-3 fields guarantee the precision of your decimal numbers. You won’t lose valuable decimal information during calculations.
Understanding COMP-3: A Real-World Analogy
Think of a COMP-3 field as a very organized filing cabinet. Each drawer in the cabinet (byte) can hold two files (digits). However, the last drawer has an additional role—it holds a special flag indicating whether the files inside represent a positive or negative value. This allows you to pack a lot of information into a small space while maintaining accuracy.
COMP-3 is a valuable tool in the COBOL programmer’s arsenal. Understanding its advantages and how it works will allow you to leverage its efficiency and precision in your COBOL applications.
How do I see the value of the field stored in comp-3 format?
COMP-3 is a data format used in older mainframe systems to represent numeric values in a compact and efficient way. The way COMP-3 stores values might seem a bit different from how you’re used to seeing numbers, but it’s really quite logical.
Imagine you have a number like 1234. In COMP-3 format, this number would be stored as a series of bytes, with each byte containing two “nibbles,” or half-bytes. Think of it like this:
1234 is split into four digits: 1, 2, 3, and 4.
* Each digit is represented by a nibble.
* The nibbles are then arranged in a byte-by-byte order.
For example, the 1234 would be stored in COMP-3 as:
* Byte 1: 01 (representing the digit 1)
* Byte 2: 02 (representing the digit 2)
* Byte 3: 03 (representing the digit 3)
* Byte 4: 04 (representing the digit 4)
COMP-3 uses a special set of characters to represent numeric values within each nibble. These characters are typically represented as hexadecimal values. For example, the digit 1 is represented as 01 in hexadecimal.
Important Note: The most significant digit (the leftmost digit in the number) is stored in the high-order nibble of the first byte, and the least significant digit (the rightmost digit) is stored in the low-order nibble of the last byte.
Here’s a breakdown of how the bytes would be arranged for the number 1234:
Byte 1: High Nibble – 01 (representing digit 1) , Low Nibble – 02 (representing digit 2)
Byte 2: High Nibble – 03 (representing digit 3) , Low Nibble – 04 (representing digit 4)
Understanding the COMP-3 format helps you interpret the raw data you might encounter in legacy systems. It’s like deciphering a code that allows you to see the value of a field even when it’s stored in a non-standard way.
What is packed decimal in COBOL?
Packed decimal is a data type that stores numbers in a way that’s super efficient and precise. Imagine you have a number like 123.45. Instead of storing it as a regular decimal, packed decimal uses each byte (a group of 8 bits) to hold two decimal digits. So, the number 123.45 would be stored as:
12 in the first byte
34 in the second byte
05 in the third byte (with a sign indicator)
This approach makes it super efficient to store decimal numbers, especially when you’re dealing with things like financial transactions where exact precision is critical.
Think of it like this: packed decimal is like a number cruncher that keeps track of every single penny. It’s all about being accurate and precise, which is why it’s a popular choice in COBOL for working with financial data.
Now, you might be wondering about the sign indicator. It’s a special character that tells us whether the number is positive or negative. The sign is usually stored in the last half-byte of the packed decimal field. There are two standard representations of this sign. One representation uses a “C” to indicate a positive sign and a “D” to indicate a negative sign. The other uses a “F” for positive and a “X” for negative.
It’s important to remember that the packed decimal representation doesn’t have a separate mantissa and exponent, so the position of the decimal point is determined by the data type you define. This means you need to clearly specify how many digits you’ll have to the left and right of the decimal point.
So, packed decimal is a valuable tool in COBOL for storing and manipulating decimal numbers with accuracy and efficiency. It’s a favorite for financial applications and anything where precision matters.
How do you display negative numbers in COBOL?
“`COBOL
05 AMOUNT PIC S9(5)V99.
“`
This definition specifies that the variable `AMOUNT` is a signed numeric variable with a maximum of five digits before the decimal point and two digits after. The “S” in the picture clause indicates that the variable is signed. If the value of `AMOUNT` is negative, the minus sign will be displayed before the number. If the value of `AMOUNT` is positive, a space will be displayed instead of a plus sign.
Now, let’s say you want to display a plus sign (+) for positive values instead of a space. You can do this by using a plus sign (+) in the picture clause. For example:
“`COBOL
05 AMOUNT PIC S9(5)V99+.
“`
With this definition, the plus sign (+) will be displayed if the value of `AMOUNT` is positive, and the minus sign (-) will be displayed if the value is negative.
Let me break down this concept in detail. When you use the “S” in the picture clause for a numeric variable, it essentially tells the compiler that this variable can hold both positive and negative values. The compiler will automatically display a minus sign (-) for negative values, but it will display a space for positive values. This is the default behavior.
However, if you want to show a plus sign (+) for positive values instead of a space, you have to explicitly include the plus sign (+) in the picture clause. This instructs the compiler to display a plus sign (+) for positive values and a minus sign (-) for negative values.
Let’s consider a practical example:
“`COBOL
DATA DIVISION.
WORKING-STORAGE SECTION.
01 WS-AMOUNT PIC S9(5)V99+.
PROCEDURE DIVISION.
MOVE +123.45 TO WS-AMOUNT.
DISPLAY WS-AMOUNT.
MOVE -456.78 TO WS-AMOUNT.
DISPLAY WS-AMOUNT.
STOP RUN.
“`
In this code, we have declared a variable called `WS-AMOUNT` with a picture clause of `S9(5)V99+`. When you run this code, the output will be:
“`
+123.45
-456.78
“`
This clearly demonstrates how the plus sign (+) is displayed for positive values and the minus sign (-) is displayed for negative values.
This is how you can control the display of negative numbers in COBOL. By understanding how the picture clause works with signed numeric variables, you can ensure your code produces outputs that are both clear and accurate.
What is the format of comp in COBOL?
Let’s break this down:
Packed Decimal: Think of it like a compact way to store numbers. Instead of using a whole byte for each digit, you pack two digits into each byte.
Most Significant Half-byte: This refers to the upper four bits of a byte. In packed decimal, this half-byte always contains a zero.
Least Significant Half-byte: This refers to the lower four bits of a byte. This is where the actual digit is stored.
Example:
Let’s say you want to store the number 1234 in a COMP field. Here’s how it would look in packed decimal:
Byte 1: 01 (0 in the most significant half-byte, 1 in the least significant half-byte)
Byte 2: 23 (2 in the most significant half-byte, 3 in the least significant half-byte)
Byte 3: 40 (4 in the most significant half-byte, 0 in the least significant half-byte)
This format is efficient because it allows you to store more numbers in less space. However, you need to be aware that you can only store numbers using this format. If you need to store characters or special symbols, you’ll need to use a different data type.
Why use COMP?
The COMP data type is ideal for performing mathematical operations. It’s designed to store numbers efficiently and accurately. COBOL compilers often optimize calculations involving COMP fields, making them faster and more efficient.
In Summary:
COMP is the COBOL data type for representing numbers in packed decimal format.
* The packed decimal format stores two digits per byte, making it a space-efficient way to represent numbers.
COMP is ideal for performing mathematical operations due to its efficiency and accuracy.
What is comp 1, comp 2, and comp-3?
COMP-1 is a data type for representing integers. These integers are stored in four bytes (32 bits) and can range from -2,147,483,648 to 2,147,483,647.
COMP-2 is a data type for representing floating-point numbers, which are numbers with a decimal point. Think of these numbers as “long floating-point” numbers. They are stored in eight bytes (64 bits) and allow for a greater range and precision than COMP-1.
COMP-3 is a data type used to represent decimal numbers, which are numbers with a decimal point. These numbers are stored internally using a special format that allows for precise representation of fractions and decimals.
So, the key takeaway is that these three data types represent numbers, but in different ways:
COMP-1: whole numbers
COMP-2: long floating-point numbers with a decimal point
COMP-3: decimal numbers internally stored with precision
Now, let’s dig a bit deeper into COMP-3 to help you understand its importance.
COMP-3 is a great choice for representing decimal numbers because it ensures accurate calculations with decimals. If you work with monetary amounts, for example, where precision is crucial, COMP-3 is the perfect data type to use. This format avoids the rounding errors that can occur with floating-point numbers.
Let’s illustrate with a simple example. Imagine you’re calculating a discount on a product that costs $10.00. You want to apply a 10% discount.
* If you use COMP-2, the calculation could potentially introduce rounding errors, leading to a slightly inaccurate discount amount.
* With COMP-3, the calculation is exact and preserves the precision of the decimal values, ensuring you get the correct discounted price.
In a nutshell, COMP-3 is an invaluable tool for working with decimal numbers, particularly when accuracy and precision are essential.
What is the difference between comp and COMP3?
COMP signifies a binary format for storing numbers. This means that the numbers are represented using only ones and zeros (bits). This is a very efficient format for storing numbers, as it uses less space than other formats.
COMP3 on the other hand, stands for packed decimal. Here, numbers are stored in a packed decimal format. This format uses a single byte to store two digits. The advantage of packed decimal is that it allows for more precise arithmetic operations. This is especially relevant for financial applications where rounding errors can have a significant impact.
Think of COMP as storing the essence of a number in a compact, binary form. COMP3 allows for more detail, like representing fractions or specific decimal places, making it ideal for calculations involving money.
Imagine you want to store a number like 1234.
COMP might represent it as a series of bits like 0000 1001 0110 0100.
COMP3 would store it as 01 23 40, with each pair of digits represented within a single byte.
This difference in representation can have implications for data storage and processing efficiency. While COMP is efficient, COMP3 provides greater precision. The choice between COMP and COMP3 is often driven by the specific requirements of the application and the trade-off between space efficiency and precision.
What is the storage of comp in COBOL?
You have three choices for storing integers using COMP: half word, full word, and double word. Think of these as different-sized containers for your numbers.
Half word: Holds integers in 2 bytes, perfect for smaller numbers.
Full word: Stores integers in 4 bytes, suitable for a wider range of values.
Double word: Utilizes 8 bytes for the largest integers.
But there’s a twist! The PICTURE clause, which defines the format of your data, plays a role too. The number of decimal digits you declare in the PICTURE clause influences the storage size, even if you specify COMP.
Let’s break this down:
PICTURE clause with no decimal digits: The storage size is determined by the COMP option (half word, full word, or double word).
PICTURE clause with decimal digits: COMP will choose the smallest storage option that can accommodate the number of digits specified. For example, if you have a PICTURE clause with 4 decimal digits, COMP will select full word storage, even if a smaller option could theoretically work.
Think of it like packing boxes. If you have a box that can fit a specific number of items, you’ll choose the smallest box that can hold them all, even if there’s space left over. This ensures your program uses the most efficient storage possible.
In short, COMP offers a way to optimize your integer storage in COBOL. It allows you to balance memory usage with the range of values you need to represent, making your code run smoothly and efficiently.
How many bytes is COMP3?
COMP3 is a packed decimal format, which means it stores data in a very compact way. It utilizes one digit/ half byte of storage space, making it incredibly efficient for storing decimal values. This means that if you have a value like 1234, it will only take up 4 bytes of storage space.
Now, let’s explore this further:
Understanding the Packed Decimal Concept
Imagine you have a number like 1234. In a traditional format, this would require 4 bytes of storage, one for each digit. But COMP3 takes a different approach – it packs the data.
How it works:
– Binary Representation: Each digit is represented in a binary format using 4 bits (half a byte).
– Packing: The binary representations of the digits are packed together, sequentially.
– Efficiency: This packing allows for storing the same number (1234) in just 4 bytes, saving space and enhancing storage efficiency.
Practical Example:
– The number 1234 in COMP3 would look like this:
– 0001 0010 0011 0100
Key Points
– COMP3 is highly efficient for storing decimal values as it significantly reduces storage space requirements compared to traditional methods.
– Each digit occupies half a byte (4 bits), and it packs these digits together, leading to very compact storage.
In Summary:
COMP3 is a powerful format when dealing with decimal values. Its packed decimal nature provides significant storage efficiency without compromising data integrity. By understanding how COMP3 operates, you can optimize your data storage strategies and save precious space.
See more here: How Do I See The Value Of The Field Stored In Comp-3 Format? | How To Convert Comp 3 To Readable Format In Cobol
How to define a comp-3 variable in COBOL program?
For example:
“`cobol
01 WS-PDN PIC 9(5) USAGE IS COMP-3.
“`
In this snippet, WS-PDN is a variable that can hold a five-digit decimal number. It’s stored in a special packed format, which saves space in memory. And the best part? You can assign values to a COMP-3 variable just like you would with any other numeric variable in COBOL.
So, what exactly is this “packed format”? Think of it like a clever way to store numbers. Instead of using a full byte for each digit, COMP-3 packs two digits into a single byte, with the sign of the number tucked into the rightmost nibble. This means you can store twice the amount of data in the same space. COMP-3 is particularly useful when you’re dealing with large amounts of numeric data, since it helps to optimize your program’s performance and memory consumption.
Here’s a more detailed breakdown of how COMP-3 packing works:
– Each byte (8 bits) can store two decimal digits, along with the sign of the number.
– The leftmost four bits of the byte store the first digit, the next four bits store the second digit, and the last nibble (four bits) stores the sign.
– A “C” in the sign position indicates a positive value, while an “F” indicates a negative value.
For instance, let’s say you have the number 12345. Here’s how it would be stored in a COMP-3 variable:
– The first byte would hold 12 (leftmost nibble) and 34 (rightmost nibble).
– The second byte would hold 5 (leftmost nibble) and C (rightmost nibble), representing a positive sign.
Understanding this packed format can be helpful when working with COMP-3 variables, especially when debugging or performing calculations. It’s a powerful technique that can significantly enhance your COBOL program’s efficiency.
What are the different formats of COBOL sign?
COMP format stores the sign in the most significant bit, which is the leftmost bit of the data representation. This means the sign is directly integrated into the numerical value itself. This is efficient in terms of storage and processing, as it doesn’t require any extra space for the sign.
COMP-3 (also known as packed decimal) represents the sign in the last nibble, which is a group of four bits. The sign is stored in the rightmost half of the last byte. This format offers a balance between efficiency and readability.
DISPLAY format uses the rightmost byte, storing the sign along with the rightmost digit of the field. This format is convenient for human readability, as the sign is explicitly visible within the data. However, it takes up more space in memory compared to COMP and COMP-3.
Delving Deeper
Now, let’s dive a little deeper into how these formats actually represent the sign. In COMP, a 0 in the most significant bit indicates a positive number, while a 1 signifies a negative number. This approach is often seen in two’s complement representation, a common method for representing negative numbers in computer systems.
COMP-3 uses a slightly different approach. The sign is encoded within the last nibble using a specific set of values. A value of C (hexadecimal) signifies a positive number, while D represents a negative number.
DISPLAY, as mentioned, simply stores the sign character alongside the data. The sign is typically a + or – character.
Here’s an example to illustrate the difference:
Assume we have a value of 123.45:
COMP: This would be stored as a binary representation with the sign bit set to 0.
COMP-3: The sign would be encoded as a ‘C’ (hexadecimal) in the last nibble, and the remaining nibbles would represent the digits.
DISPLAY: The sign would be a ‘+’ character stored along with the digits, resulting in a string like “+123.45”.
Understanding these different formats helps you choose the most appropriate one for your specific needs. If efficiency is paramount, COMP might be the best choice. If you prioritize readability or need to work with character-based input, DISPLAY could be more suitable. COMP-3 strikes a balance, offering good performance while still being reasonably easy to work with.
How do I convert a COBOL file to a CSV file?
1. Mainframe Conversion:
The most straightforward method is to perform the conversion directly on the mainframe using COBOL. This usually involves creating a new COBOL program that reads the original COBOL file and writes the data to a CSV file. This method is usually the most efficient and reliable, especially if you’re working within a mainframe environment.
2. External Tools:
If you need to convert the file outside of the mainframe, you can leverage tools like coboltocsv. These tools utilize COBOL copybooks to define the structure of the data in your COBOL file. The copybook acts like a blueprint, telling the tool how to interpret the data and format it into CSV.
3. Handling Comp-3 Data:
You asked about comp-3 data, and that’s a great question! Comp-3 is a COBOL data type used for storing packed decimal numbers. These numbers are represented in a compressed format, meaning they take up less space than standard decimal numbers. To convert comp-3 data, you’ll need to unpack it into a standard decimal format, which can be done with a variety of methods:
COBOL Programs: You can write a COBOL program to handle the unpacking.
External Tools: Many conversion tools like coboltocsv have built-in support for handling comp-3 data.
Let’s dive into using a COBOL copybook for conversion:
A COBOL copybook is a file containing data definitions that can be included in your COBOL programs. The copybook defines the structure of your data, including things like:
Field Names: Descriptive names for each data element.
Data Types: The type of data stored in each field (e.g., comp-3, alphanumeric, etc.).
Field Lengths: The number of characters or bytes allocated to each field.
When you use a tool like coboltocsv, you provide the copybook along with the COBOL file. The tool uses the copybook to understand the structure of the data in your COBOL file, and then it generates a CSV file with the data formatted accordingly.
For example, let’s say you have a COBOL file with data about employees. The copybook might define fields like:
Employee ID (comp-3, 5 bytes)
Employee Name (Alphanumeric, 30 characters)
Department (Alphanumeric, 10 characters)
Salary (comp-3, 8 bytes)
The coboltocsv tool would use this copybook to extract the data from your COBOL file and generate a CSV file with columns for Employee ID, Employee Name, Department, and Salary.
Remember, the conversion process is highly dependent on the specific structure of your COBOL file and the chosen conversion method. However, by understanding the basic principles of COBOL data types, copybooks, and CSV formatting, you’ll be well-equipped to tackle any COBOL-to-CSV conversion project!
What if U get 000004040404 on comp-3 variable?
If you encounter 000004040404 in a COMP-3 variable, it indicates that the variable is empty. It’s like finding an empty box; there’s nothing inside.
This often happens when you’re dealing with data that comes from an external source, like a file. You might find yourself trying to read data into a table and encountering this peculiar value.
The key to understanding COMP-3 variables is their packed representation. COMP-3 is a packed decimal format, where each digit occupies half a byte. To represent a negative sign, they use a special code, which can be confusing if you’re not familiar with the format.
So what does it mean when you see 000004040404 in a COMP-3 variable?
Because the representation is packed, each byte contains two digits. You’re looking at a variable where all the digits are 0 and 4. This is the sign code for negative zero, which essentially indicates an empty value. So while you see a value, it doesn’t actually represent a valid number.
Moving Data Between COMP-3 Variables
Now, let’s address your question about moving data between COMP-3 variables. The answer is a bit complex and depends on the specific programming language or system you’re using.
Generally, you can move data between variables of different lengths. However, you must be mindful of the potential for data truncation or overflow.
For example, if you try to move data from an S9(10)V99 COMP-3 variable to an S9(07)V99 COMP-3 variable, you’re likely to lose the rightmost digits because the target variable is shorter.
It’s important to ensure that your target variable is large enough to accommodate the entire value from the source variable. If you’re unsure, you can consult the documentation for your specific programming language or system for guidelines on handling data type conversions.
Here’s an analogy: Imagine you have a box that can hold 10 items, and you want to move those items into a smaller box that can only hold 7 items. You would need to discard the extra 3 items to fit everything in the smaller box. The same concept applies to moving data between COMP-3 variables of different lengths.
Keep in mind that data truncation can lead to unexpected results, especially when dealing with financial or numerical data. It’s crucial to handle these situations carefully and ensure data integrity.
See more new information: musicbykatie.com
How To Convert Comp-3 To Readable Format In Cobol
Understanding COMP-3
First, let’s break down what COMP-3 is. It’s a COBOL data type used to store packed decimal data. Think of it as a way to squeeze more information into a smaller space. It’s great for efficiency, but it also makes the data harder to read. Essentially, COMP-3 stores a numeric value in a compressed format, where each byte (except the last) represents two decimal digits, and the last byte contains the sign and the rightmost digit.
The Need for Conversion
The reason we need to convert COMP-3 to a readable format is pretty simple. Humans like to see things in a plain, human-readable way. COMP-3 data is great for storage, but it’s not friendly when you want to display it or use it for calculations outside of your program.
Methods for Conversion
Now, let’s look at how you can convert COMP-3 data to a readable format. The best part? You can accomplish this in a few different ways:
1. Using the INSPECT Statement:
This is probably the most straightforward way. The INSPECT statement allows you to search for and replace characters within a string. Here’s a simple example:
“`cobol
DATA DIVISION.
WORKING-STORAGE SECTION.
01 WS-COMP-3 PIC S9(4) COMP-3.
01 WS-READABLE PIC X(5).
PROCEDURE DIVISION.
MOVE 1234 TO WS-COMP-3.
INSPECT WS-COMP-3 CONVERTING ‘ ‘ TO ‘0’
REPLACING ALL ‘C’ BY ‘ ‘
REPLACING ALL ‘D’ BY ‘ ‘
REPLACING ALL ‘F’ BY ‘-‘.
MOVE WS-COMP-3 TO WS-READABLE.
DISPLAY WS-READABLE.
STOP RUN.
“`
In this example, we take the COMP-3 value in WS-COMP-3 and use INSPECT to convert it to a readable format in WS-READABLE. The CONVERTING clause is used to replace any spaces with zeros, and the REPLACING clauses handle the sign conversion. The ‘C’, ‘D’, and ‘F’ are characters that represent positive, negative, and zero signs, respectively.
2. Using the MOVE Statement and PICTURE Clause:
This method is a bit more direct. The key is using the correct PICTURE clause. Here’s an example:
“`cobol
DATA DIVISION.
WORKING-STORAGE SECTION.
01 WS-COMP-3 PIC S9(4) COMP-3.
01 WS-READABLE PIC 9(4).
PROCEDURE DIVISION.
MOVE 1234 TO WS-COMP-3.
MOVE WS-COMP-3 TO WS-READABLE.
DISPLAY WS-READABLE.
STOP RUN.
“`
In this case, we’re moving the COMP-3 value in WS-COMP-3 to the WS-READABLE variable. The PICTURE clause of WS-READABLE is set to 9(4), which tells COBOL to treat the value as a standard numeric value. COBOL handles the conversion automatically.
3. Using the UNSTRING Statement:
You can also utilize the UNSTRING statement, which is useful for breaking down the packed decimal value into individual digits.
“`cobol
DATA DIVISION.
WORKING-STORAGE SECTION.
01 WS-COMP-3 PIC S9(4) COMP-3.
01 WS-READABLE PIC X(5).
01 WS-DIGIT PIC 9.
PROCEDURE DIVISION.
MOVE 1234 TO WS-COMP-3.
UNSTRING WS-COMP-3 DELIMITED BY SIZE
INTO WS-DIGIT WS-DIGIT WS-DIGIT WS-DIGIT
WITH POINTER WS-POINTER
TALLYING WS-COUNT.
MOVE WS-DIGIT TO WS-READABLE(1:1).
MOVE WS-DIGIT TO WS-READABLE(2:1).
MOVE WS-DIGIT TO WS-READABLE(3:1).
MOVE WS-DIGIT TO WS-READABLE(4:1).
DISPLAY WS-READABLE.
STOP RUN.
“`
Here, we use UNSTRING to unpack the COMP-3 value into individual digits stored in WS-DIGIT. We then build the readable format in WS-READABLE.
Choosing the Right Method
You might be wondering, “Which method should I use?” It depends on your situation. The INSPECT statement is very flexible, allowing you to handle different sign representations. The MOVE statement is simpler and more efficient if you only need a basic conversion. The UNSTRING statement offers more control over individual digits.
FAQs:
Q: What if my COMP-3 data has a decimal point?
A: You’ll need to adjust your code to account for the decimal point. Use the PICTURE clause appropriately, and make sure your MOVE or INSPECT statements handle the decimal placement.
Q: What about negative numbers?
A: COMP-3 uses a sign bit to represent negative numbers. Make sure your conversion methods handle the sign correctly.
Q: Can I convert COMP-3 to a character string?
A: Yes! You can use the MOVE statement with a PICTURE clause of X to move the COMP-3 value to a character string. However, remember that this will result in the character representation of the packed decimal value, not a human-readable number.
Q: Why not just use a different data type instead of COMP-3?
A: In some cases, using COMP-3 might be unavoidable, especially when dealing with older programs or systems where space efficiency is critical.
Additional Considerations
* Performance: If you’re working with large amounts of COMP-3 data, consider the performance impact of your conversion method. The MOVE statement is generally more efficient than INSPECT or UNSTRING.
* Maintainability: Choose a method that is clear and easy to understand for future developers.
Readability: Ultimately, the goal is to make your data human-readable. Don’t overcomplicate your conversions with unnecessary steps or logic.
Let me know if you have any other questions about working with COMP-3 in COBOL. Good luck!
Converting comp-3 back to a human readable format
The best option is to do the conversion on the mainframe / Cobol and transfer a Text file. Alternatively There are projects like coboltocsv which will convert a Stack Overflow
Convert COMP-3 into readable format – IBM Mainframe Community
COBOL Programming: How do i display a comp-3 variable in readable format in COBOL. IBM Mainframe Community
COMP-3 in COBOL – GeeksforGeeks
For WS-VALC PIC S9(3) USAGE IS COMP-3, so here we can take it as (3+1)/2 which is equal to 2bytes size. Similarly, in the 3byte variable which is WS-VALD GeeksForGeeks
How to convert COMP-3 to Regular Number? – COBOL General
The comp-3 format means: x’12345c’ for +12345. x’12345d’ for -12345. x’12345f’ for 12345. so you can compare the valid hexadecimal values to find out what Tek-Tips Forums
COBOL COMP-3 | Packed-decimal
To define a COMP-3 variable in the COBOL program, we use the USAGE IS COMP-3 clause in the DATA DIVISION. For example – 01 WS-PDN PIC 9(5) USAGE IS COMP-3. Mainframestechhelp
Formats for numeric data – IBM
As with external decimal numbers, external floating-point numbers have to be converted (by the compiler) to an internal representation of their numeric value before they can be IBM
Convert packed decimal (comp-3) to readable format
I have an input file with Comp-3 data from column 113-117 with picture clause S9(10)V99. Total lenght of input file is 292 bytes. My requirement is to convert comp-3 mvsforums.com
COBOL – SIGN STORED IN COMP, COMP-3 AND DISPLAY
Sign is stored in different formats as shown below. COMP : IN THE MOST SIGNIFICANT BIT. COMP-3 : IT IS STORED IN THE LAST NIBBLE. DISPLAY : THE F1 for Mainframe
Moving Numeric to COmp 3 – IBM Cobol – IBM Mainframe Forum
Assuming that the PIC X variable does have nothing but numeric digits, your best bet is a two-step move: move the PIC X to USAGE DISPLAY variable, then move ibmmainframeforum.com
Cobol: Zoned Decimal And Packed Decimal
Converting Numeric Formats (Zd To Pd, Zd To Bi And Reverse)
Cobol Comp, Comp1, Comp2, Comp3 Variables
Cobol Usage Computational Clauses (Comp \U0026 Comp-3)
Viewing Comp Data | Comp-1 | Comp-2 | Comp-3
Cobol Lesson 120 – Comp-3 Packed Decimal Numerics
Convert Mainframe Cobol Ebcdic Data To Xml, Csv Or Tab In 60 Seconds
Cobol Comp, Comp-1, Comp-2 And Comp-3
Link to this article: how to convert comp 3 to readable format in cobol.
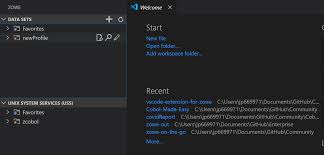
See more articles in the same category here: https://musicbykatie.com/wiki-how/